ジオメトリとマテリアルから作成したメッシュをシーンに追加する。
App.tsx
import React from 'react'
import { Canvas } from '@react-three/fiber';
import { PerspectiveCamera, OrbitControls } from '@react-three/drei';
import GlobalStyle from './components/GlobalStyle';
import Container from './components/Container';
import Plane from './components/Plane';
import Cube from './components/Cube';
const App = () => {
const width: number = window.innerWidth;
const height: number = window.innerHeight;
return (
<React.Fragment>
<GlobalStyle />
<Container>
<Canvas>
<PerspectiveCamera makeDefault fov={60} aspect={width/height} position={[15,15,15]} />
<Plane />
<Cube />
<axesHelper args={[12]} />
<OrbitControls enablePan={false} enableZoom={false} enableRotate={false} />
</Canvas>
</Container>
</React.Fragment>
);
}
export default App;
GlobalStyle.tsx
import { createGlobalStyle } from 'styled-components';
const GlobalStyle = createGlobalStyle`
body {
margin: 0;
}
`;
export default GlobalStyle;
Container.tsx
import styled from 'styled-components';
const Container = styled.div`
width: 100vw;
height: 100vh;
background-color: black;
`;
export default Container;
Plane.tsx
import React from 'react';
const Plane: React.FC = () => {
return (
<mesh>
<planeGeometry attach="geometry" args={[10,10]} />
<meshBasicMaterial attach="material" />
</mesh>
);
}
export default Plane;
Cube.tsx
import React from 'react';
const Cube: React.FC = () => {
return (
<mesh>
<boxGeometry attach="geometry" args={[1,1,1]} />
<meshNormalMaterial attach="material" />
</mesh>
);
}
export default Cube;
今回は、以下のように出力される。
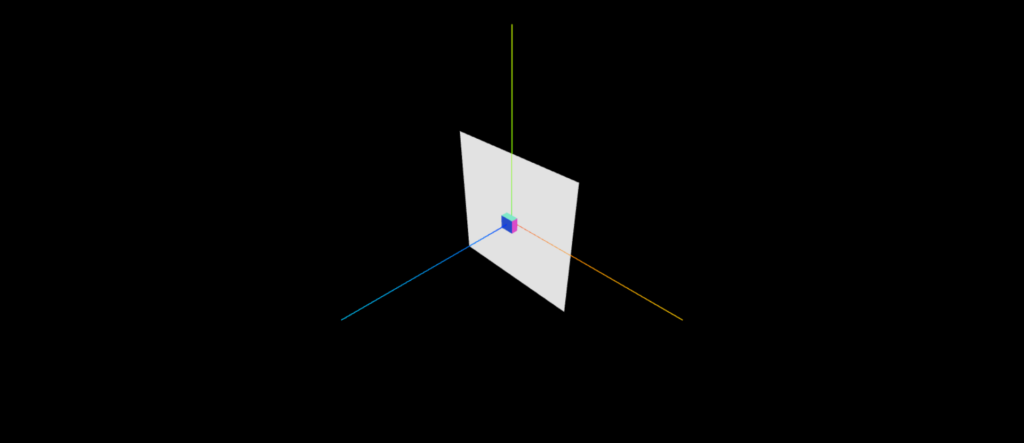