Canvasに迷路を描画する。
Canvas.tsx
import React, { useEffect } from 'react';
import p5 from 'p5';
import Maze from './maze';
const sketch = (p: p5) => {
const board_w: number = 450, board_h: number = 450, cell_space: number = 5;
let space_w: number, space_h: number;
let maze1: Maze, position: number[];
p.setup = () => {
p.createCanvas(640, 480);
space_w = (p.width-board_h)/2;
space_h = (p.height-board_h)/2;
maze1 = new Maze(p, 15, 15);
maze1.set_maze_boutaoshi();
position = maze1.start;
}
p.draw = () => {
p.background('black');
p.noFill();
p.stroke('white');
p.rect(space_w, space_h, board_w, board_h);
for (let i = 1; i < 3; i++) {
p.line(space_w, i*(board_h/3)+space_h, p.width-space_w, i*(board_h/3)+space_h);
p.line(i*(board_w/3)+space_w, space_h, i*(board_w/3)+space_w, p.height-space_h);
}
p.fill('white');
p.ellipse(p.width/2, p.height/2, (board_w/3)-(cell_space*2),(board_h/3)-(cell_space*2));
for (let y = 0; y < 3; y++) {
for (let x = 0; x < 3; x++) {
if (maze1.maze[y+position[1]-1][x+position[0]-1] === 1) {
p.rect(x*(board_w/3)+space_w+cell_space,y*(board_h/3)+space_h+cell_space,(board_w/3)-(cell_space*2),(board_h/3)-(cell_space*2));
}
}
}
}
}
const Canvas: React.FC = () => {
useEffect(() => {
new p5(sketch)
})
return (
<React.Fragment>
</React.Fragment>
);
}
export default Canvas;
import p5 from 'p5';
type maze = number[][] | string[][];
type binary = 0 | 1;
class Maze {
p: p5;
PATH: number;
WALL: number;
width: number;
height: number;
maze: maze = [];
dist: number[][] = [];
start: number[] = [];
goal: number[] = [];
constructor(p: p5, width: number, height: number, seed: number = 0) {
this.p = p;
this.PATH = 0;
this.WALL = 1;
this.width = width;
this.height = height;
if (this.width < 5 || this.height < 5) {
return;
}
if (this.width%2 === 0) {
this.width++;
}
if (this.height%2 === 0) {
this.height++;
}
this.maze = [...Array(this.height)].map(() => Array(this.width).fill(0));
this.dist = [...Array(this.height)].map(() => Array(this.width).fill(-1));
this.start = [1, 1];
this.goal = [this.width-2, this.height-2];
p.randomSeed(seed);
}
set_outer_wall(): maze {
for (let y = 0; y < this.height; y++) {
for (let x = 0; x < this.width; x++) {
if (x === 0 || y === 0 || x === this.width-1 || y === this.height-1) {
this.maze[y][x] = this.WALL;
}
}
}
return this.maze;
}
set_inner_wall(): maze {
for (let y = 2; y <= this.height-3; y+=2) {
for (let x = 2; x <= this.width-3; x+=2) {
this.maze[y][x] = this.WALL;
}
}
return this.maze;
}
set_maze_boutaoshi(): maze {
let cell_x: number, cell_y: number, direction: number;
this.set_outer_wall();
this.set_inner_wall();
for (let y = 2; y <= this.height-3; y+=2) {
for (let x = 2; x <= this.width-3; x+=2) {
while (true) {
cell_x = x;
cell_y = y;
if (y === 2) {
direction = this.p.floor(this.p.random(4));
} else {
direction = this.p.floor(this.p.random(3));
}
if (direction === 0) {
cell_x += 1;
} else if (direction === 1) {
cell_y += 1;
} else if (direction === 2) {
cell_x -= 1;
} else if (direction === 3) {
cell_y -= 1;
}
if (this.maze[cell_y][cell_x] !== this.WALL) {
this.maze[cell_y][cell_x] = this.WALL;
break;
}
}
}
}
return this.maze;
}
set_start_goal(start: number[], goal: number[]): maze {
if (this.maze[start[1]][start[0]] === this.PATH) {
this.start = start;
}
if (this.maze[goal[1]][goal[0]] === this.PATH) {
this.goal = goal;
}
return this.maze;
}
set_dist_bfs(flag: boolean | binary = false): number[][] {
let queue: number[][] = [], point: number[];
this.dist[this.start[1]][this.start[0]] = 0;
queue.push(this.start);
while (queue.length > 0) {
point = queue.shift()!;
for (let x of [[0,-1],[1,0],[0,1],[-1,0]]) {
if (this.maze[point[1]+x[1]][point[0]+x[0]] === 0 && this.dist[point[1]+x[1]][point[0]+x[0]] === -1) {
this.dist[point[1]+x[1]][point[0]+x[0]] = this.dist[point[1]][point[0]] + 1;
queue.push([point[0]+x[0],point[1]+x[1]]);
}
if (flag !== true && flag !== 1) {
if (point[0]+x[0] === this.goal[0] && point[1]+x[1] === this.goal[1]) {
queue = [];
break;
}
}
}
}
return this.dist;
}
set_dist_dfs(flag: boolean | binary = false): number[][] {
let stack: number[][] = [], point: number[];
this.dist[this.start[1]][this.start[0]] = 0;
stack.push(this.start);
while (stack.length > 0) {
point = stack.pop()!;
for (let x of [[0,-1],[1,0],[0,1],[-1,0]]) {
if (this.maze[point[1]+x[1]][point[0]+x[0]] === 0 && this.dist[point[1]+x[1]][point[0]+x[0]] === -1) {
this.dist[point[1]+x[1]][point[0]+x[0]] = this.dist[point[1]][point[0]] + 1;
stack.push([point[0]+x[0],point[1]+x[1]]);
}
if (flag !== true && flag !== 1) {
if (point[0]+x[0] === this.goal[0] && point[1]+x[1] === this.goal[1]) {
stack = [];
break;
}
}
}
}
return this.dist;
}
set_shortest_path(): maze {
let point: number[] = this.goal;
const x: number[][] = [[0,-1],[1,0],[0,1],[-1,0]];
this.maze[point[1]][point[0]] = '*';
while (this.dist[point[1]][point[0]] > 0) {
for (let i = 0; i < x.length; i++) {
if (this.dist[point[1]][point[0]]-this.dist[point[1]+x[i][1]][point[0]+x[i][0]] === 1) {
if (this.dist[point[1]][point[0]] > 0) {
this.maze[point[1]+x[i][1]][point[0]+x[i][0]] = '*';
point = [point[0]+x[i][0],point[1]+x[i][1]];
}
}
}
}
return this.maze;
}
print_maze(): void {
let arr: string;
this.maze[this.start[1]][this.start[0]] = 'S';
this.maze[this.goal[1]][this.goal[0]] = 'G';
for (let row of this.maze) {
arr = '';
for (let cell of row) {
if (cell === this.WALL) {
arr += '#';
} else if (cell === this.PATH) {
arr += ' ';
} else if (cell === 'S') {
arr += 'S';
} else if (cell === 'G') {
arr += 'G';
} else if (cell === '*') {
arr += '*';
}
}
console.log(arr);
}
}
print_dist(): void {
let arr: string;
for (let row of this.dist) {
arr = '';
for (let cell of row) {
if (cell === -1) {
if (Math.max.apply(null, Array.prototype.concat.apply([], this.dist)) === -1) {
arr += '#';
} else {
for (let i = 0; i < this.p.str(Math.max.apply(null, Array.prototype.concat.apply([], this.dist))).length; i++) {
arr += '#';
}
}
} else {
if (this.p.str(cell).length === this.p.str(Math.max.apply(null, Array.prototype.concat.apply([], this.dist))).length) {
arr += this.p.str(cell);
} else {
for (let i = 0; i < this.p.str(Math.max.apply(null, Array.prototype.concat.apply([], this.dist))).length-this.p.str(cell).length; i++) {
arr += ' ';
}
arr += this.p.str(cell);
}
}
}
console.log(arr);
}
}
}
export default Maze;
今回は、以下のように出力される。
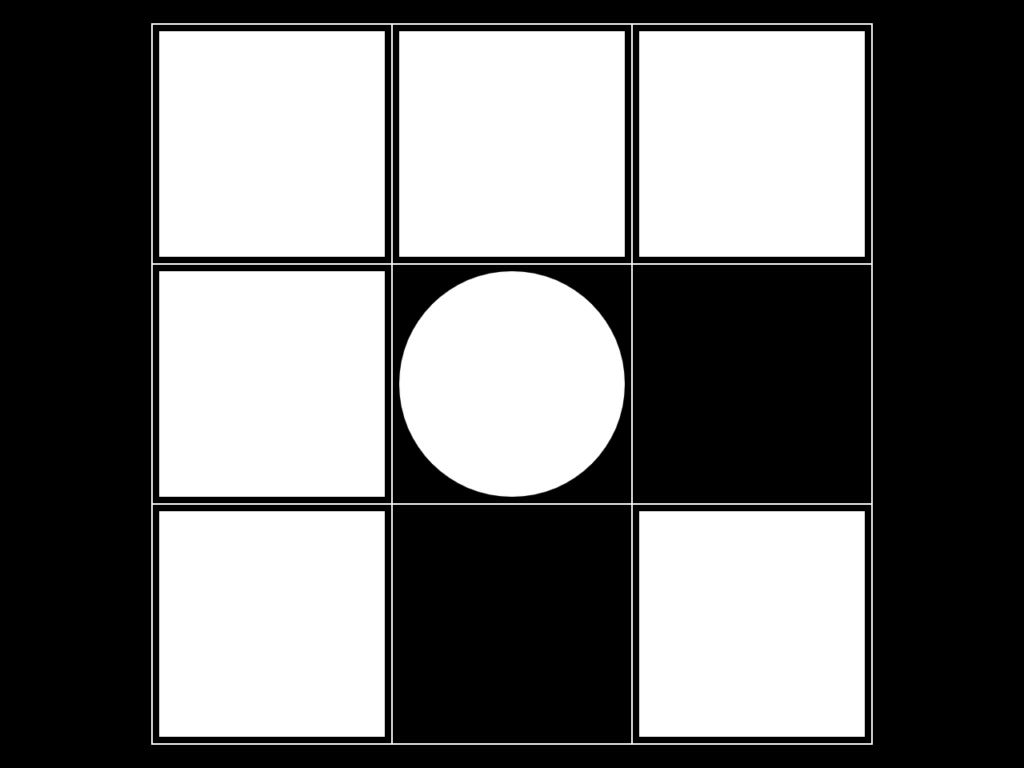