探索していない通路を「-1」として表現して
スタートからゴールに到達するまで幅優先探索で迷路を探索し、
探索した通路はスタートからの距離に数値を置き換える。
using System;
using System.Collections.Generic;
public class Maze {
public const int PATH = 0;
public const int WALL = 1;
public int width;
public int height;
public int[,] maze;
public int[,] dist;
public int[] start;
public int[] goal;
private Random random;
public Maze(int width, int height, int seed = 0) {
this.width = width;
this.height = height;
if (this.width < 5 || this.height < 5) {
Environment.Exit(0);
}
if (this.width % 2 == 0) {
this.width++;
}
if (this.height % 2 == 0) {
this.height++;
}
this.maze = new int[this.height,this.width];
for (int y = 0; y < this.height; ++y) {
for (int x = 0; x < this.width; ++x) {
this.maze[y,x] = Maze.PATH;
}
}
this.dist = new int[this.height,this.width];
for (int y = 0; y < this.height; ++y) {
for (int x = 0; x < this.width; ++x) {
this.dist[y,x] = -1;
}
}
this.start = new int[] {1, 1};
this.goal = new int[] {this.width-2, this.height-2};
this.random = new Random(seed);
}
public int[,] set_outer_wall() {
for (int y = 0; y < this.height; ++y) {
for (int x = 0; x < this.width; ++x) {
if (x == 0 || y == 0 || x == this.width-1 || y == this.height-1) {
this.maze[y,x] = Maze.WALL;
}
}
}
return this.maze;
}
public int[,] set_inner_wall() {
for (int y = 2; y < this.height-1; y += 2) {
for (int x = 2; x < this.width-1; x += 2) {
this.maze[y,x] = Maze.WALL;
}
}
return this.maze;
}
public int[,] set_maze_boutaoshi() {
int wall_x;
int wall_y;
int direction;
this.set_outer_wall();
this.set_inner_wall();
for (int y = 2; y < this.height-1; y += 2) {
for (int x = 2; x < this.width-1; x += 2) {
while (true) {
wall_x = x;
wall_y = y;
if (y == 2) {
direction = random.Next(0,4);
} else {
direction = random.Next(0,3);
}
switch (direction) {
case 0:
wall_x += 1;
break;
case 1:
wall_y +=1;
break;
case 2:
wall_x -=1;
break;
case 3:
wall_y -=1;
break;
}
if (this.maze[wall_y,wall_x] != Maze.WALL) {
this.maze[wall_y,wall_x] = Maze.WALL;
break;
}
}
}
}
return this.maze;
}
public int[,] set_start_goal(int[] start, int[] goal) {
if (this.maze[start[1],start[0]] == Maze.PATH) {
this.start = start;
}
if (this.maze[goal[1],goal[0]] == Maze.PATH) {
this.goal = goal;
}
return this.maze;
}
public int[,] set_dist_bfs(bool flag = false) {
int[] point;
int[,] x = new int[,] {{0,-1},{1,0},{0,1},{-1,0}};
Queue<int[]> queue = new Queue<int[]>();
this.dist[this.start[1],this.start[0]] = 0;
queue.Enqueue(this.start);
while (queue.Count > 0) {
point = queue.Dequeue();
for (int i = 0; i < x.GetLength(0); ++i) {
if (this.maze[point[1]+x[i,1],point[0]+x[i,0]] == 0 && this.dist[point[1]+x[i,1],point[0]+x[i,0]] == -1) {
this.dist[point[1]+x[i,1],point[0]+x[i,0]] = this.dist[point[1],point[0]] + 1;
queue.Enqueue(new int[] {point[0]+x[i,0],point[1]+x[i,1]});
}
if (flag != true) {
if (point[0]+x[i,0] == this.goal[0] && point[1]+x[i,1] == this.goal[1]) {
queue.Clear();
break;
}
}
}
}
return this.dist;
}
public int[,] set_shortest_path() {
int[] point;
int[,] x = new int[,] {{0,-1},{1,0},{0,1},{-1,0}};
point = this.goal;
this.maze[point[1],point[0]] = '*';
while (this.dist[point[1],point[0]] > 0) {
for (int i = 0; i < x.GetLength(0); ++i) {
if (this.dist[point[1],point[0]]-this.dist[point[1]+x[i,1],point[0]+x[i,0]] == 1) {
if (this.dist[point[1],point[0]] > 0) {
this.maze[point[1]+x[i,1],point[0]+x[i,0]] = '*';
point = new int[] {point[0]+x[i,0],point[1]+x[i,1]};
}
}
}
}
return this.maze;
}
public void print_maze() {
this.maze[this.start[1],this.start[0]] = 'S';
this.maze[this.goal[1],this.goal[0]] = 'G';
for (int y = 0; y < this.maze.GetLength(0); ++y) {
for (int x = 0; x < this.maze.GetLength(1); ++x) {
if (this.maze[y,x] == Maze.WALL) {
Console.Write('#');
} else if (this.maze[y,x] == Maze.PATH) {
Console.Write(' ');
} else if (this.maze[y,x] == 'S') {
Console.Write('S');
} else if (this.maze[y,x] == 'G') {
Console.Write('G');
} else if (this.maze[y,x] == '*') {
Console.Write('*');
}
}
Console.WriteLine();
}
}
public static void Main() {
Maze maze1 = new Maze(15, 15);
maze1.set_maze_boutaoshi();
maze1.set_start_goal(new int[] {9, 5}, new int[] {9, 11});
maze1.set_dist_bfs();
maze1.set_shortest_path();
maze1.print_maze();
}
}
今回は、以下のように出力される。
###############
# # # # #
### # ### ### #
# #
# ### ##### # #
# # ****S# # #
# ###*####### #
# # *** # #
##### #*##### #
# #* # #
# #####*### # #
# # **G# # #
### ### ##### #
# # # #
###############
参考
幅優先探索 - Algoful
幅優先探索(BFS)とは隣接するノードを優先して探索するアルゴリズムです。キュー(FIFO)を利用して探索を行います。迷路探索のシミュレーションで視覚的に理解できます。C#の実装サンプルがあります。
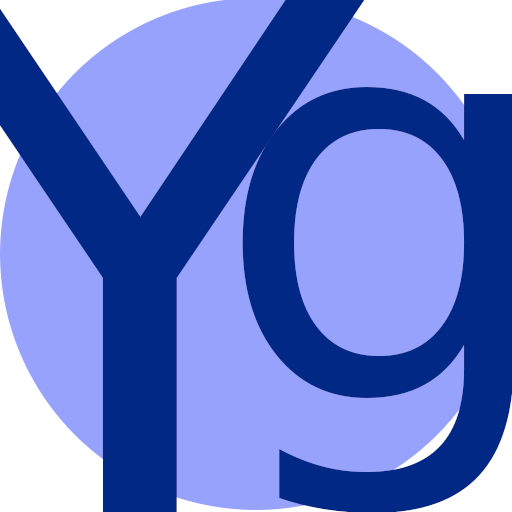
[Python] 幅優先探索で迷路を解く
幅優先探索 幅優先探索(Breadth First Search)とは、グラフを始点から近い順に1つずつ調べて…
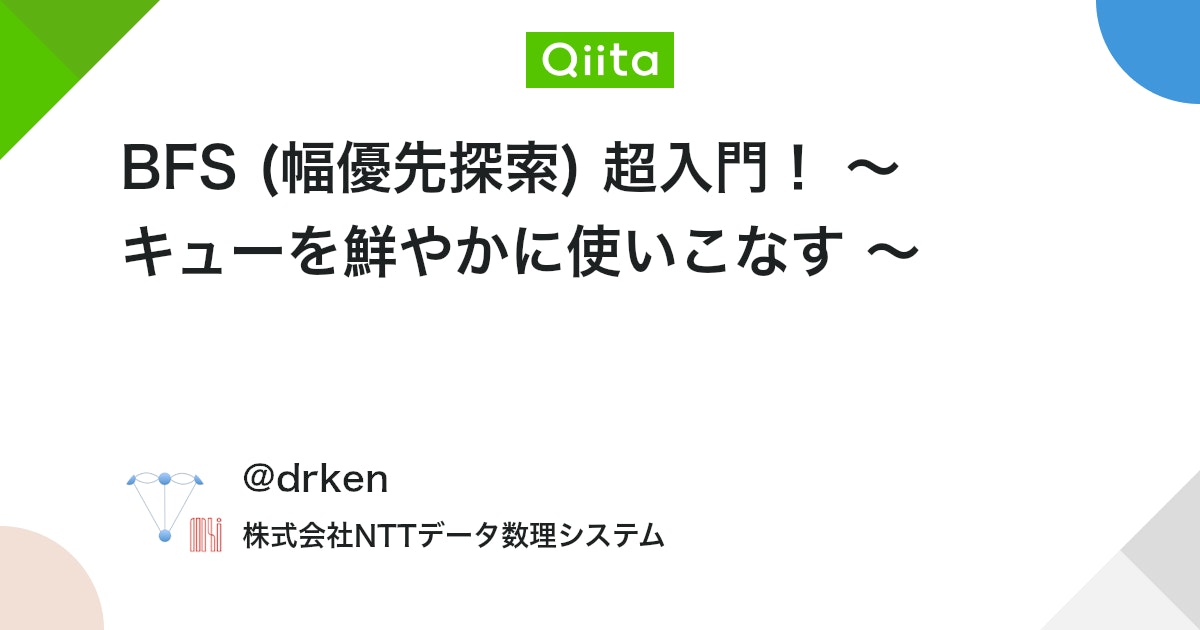
BFS (幅優先探索) 超入門! 〜 キューを鮮やかに使いこなす 〜 - Qiita
0. はじめに メジャーなグラフ探索手法には深さ優先探索 (depth-first search, DFS) と幅優先探索 (breadth-first search, BFS) とがあります1。このうち DFS については DFS (深さ...